|
SprayQc - plugin development
SprayQc provides an extensible environment for plugins. In this manual it is explained how to develop a plugin in the C# .NET framework.
Globally, plugin development involves implementation of the interface 'IPlugin' defined in the library 'SprayQc.interfaces.dll'. This
interface defines all the calls that need to be implemented so the SprayQc container can communicate with the plugin. Additionally, it
provides access to the SprayQc container class for the plugin to interact with.
The UML diagram above gives a global overview of the setup. A more comprehensive view can be found
here. The UML diagram provides a complete overview of all the classes and enumerated types involved
in plugin development.
- IPlugin
This interface provides the definition for a plugin that is used within the SprayQc framework.
- ISprayQc
This interface provides the definition of the SprayQc framework that can be used by a plugin to gather information.
- IRawFillename
This interface provides the definition for information pertaining to the currently recorded raw file. As the SprayQc application centers around raw-files, this is some of the most important information and is passed through almost all callback methods.
- INotification
This interface defines all the information required to make a notification to the operator.
- PluginType
Definition of the different roles a plugin can assume within the framework.
- CatastrophicError
Definition off whether the catastrophic error is reported or resolved.
- MessageType
Plugins can report messages to the message box in the SprayQc application. This does not trigger any notifications to the operator and as such can be used for non-critical updates. The type (error, warning, update) is specified here.
- MeasurementState
This defines the different states a measurement can be in. Idling in this case means no measurement and further there are Init, SampleLoad, PrepareMeasurement, Gradient and Washout. These states can be requested from the SprayQc application and reacted to by the plugins.
This first step is to link your project to the DLL's of SprayQc. In Visual studio this can be done by right-clicking on the 'References'
in the project tree and selecting 'Add Reference'. Select the tab 'Browse' in the dialog. In this dialog select 'SprayQc.interfaces.dll'
and 'SprayQc.utils.dll' and press OK.
When this is done you can proceed to create a class (e.g. ExampleDevice). When the class has been created, the following namespaces are
required. With these the new class can inherit from IPlugin.
using System;
using System.Drawing;
using System.Windows.Forms;
using SprayQc.interfaces;
public class ExampleDevice : IPlugin
{
public ExampleDevice(ISprayQc sprayqc)
: base(sprayqc)
{
}
By inheriting from IPlugin a special constructor is required, which is passed the instance of the SprayQc application. The base constructor
stores this instance in the protected member 'ISprayQcInstance' for future reference by the plugin.
Additionally a number of functions are required to be implemented. The first section deal with the window environment in which the plugin needs
to play a role. The first if these function is the Dispose, which is called when the application closes and all allocated resources need to be
released. In this simple example no resources are present and the function is empty.
#region IPlugin overrides
public override void Dispose()
{
}
The GetName function is used by SprayQc to give a name to the plugin in the plugin list.
public override string GetName()
{
return "Example";
}
The GetPluginType function is used by SprayQc to determine the role of the plugin. For this example it is regular, since it has no need of
further rights. In the case of a chromatographic device, which is central to the time management of the whole application, the type is
MeasurementManagement. In the case of a plugin that notifies the operator it is Notification.
public override PluginType GetPluginType()
{
return PluginType.Regular;
}
SprayQc shows a plugin specific plugin in the plugin list, which can be specified through this function. The resource is a specific class type
which can be made in Visual Studio.
public override Bitmap GetIcon()
{
return Example_Resource.application;
}
Each plugin can define multiple views (or UserControl classes in the .NET framework) to display settings, overview information, etc. With this
function the views can be exported to the SprayQc application, which will be shown in the plugin tree-view below the plugin name. The user control
must define a unique name in their implementation.
In the example, the _ctrlOverview is an instance of the CtrlOverview class described later.
public override UserControl[] GetControls()
{
return new UserControl[]{
_ctrlOverview
};
}
The monitoring functions are used to indicate that the SprayQc application is starting or stopping monitoring the peripheral equipment. For
the example we’re not actually monitoring any equipment, but here would be the place to connect to the equipment and start reading out data.
public override void MonitoringStart()
{
}
public override void MonitoringFinish()
{
}
The pending function is used to indicate that a measurement is pending for which the filename is already known. This can be used by the plugin
to prepare for the monitoring (ie by opening up a filestream in the auxiliary directory, for which the name can be found in the rawFile instance).
public override void MeasurementPending(IRawFilename rawFile)
{
}
The start function is used to indicate that the measurement has actually begun. From this point the plugin can monitor the incoming data and
store it in the auxiliary directory.
public override void MeasurementStart(IRawFilename rawFile)
{
}
The finish function is used to indicate that the measurement has finished. From this point all the filestreams can be closed and stopped to
monitor the incoming data.
public override void MeasurementFinish(IRawFilename rawFile)
{
}
The notify function should only be implemented by plugins that take on the role of Notification (See GetPluginType). This is where the SprayQc
application passes the messages that need to be send to the operator.
public override void Notify(IRawFilename rawFile, string subject, string body, string[] attachments)
{
throw new NotImplementedException();
}
This method is called when one of the plugins throws a catastrophic error. This can be used to shut down operation of the device to prevent
damage or waste of samples.
public override void OnCatastrophicError(CatastrophicError error)
{
}
Here an empty example of a view is given. The important point is that the Name member should be set to a unique name.
using System.Windows.Forms;
public partial class CtrlOverview : UserControl
{
public CtrlOverview()
{
InitializeComponent();
Name = "Example overview";
}
}
Accessing SprayQc information |
---|
Besides setting up the callbacks, the plugin also needs access to central information provided by the ISprayQcApp member. This paragraph
describes the function calls that can be used to get to this information.
When a plugin with the role MeasurementManagement triggers the MeasurementStart, this will return true. This can be used by plugins to
determine whether a measurement is ongoing, without the need of keep track of this itself. This does not convey information about the stage
at which the measurement is at this point.
bool IsMeasuring();
Retrieves the current state of the measurement. Idling in this case means no measurement and further there are Init, SampleLoad,
PrepareMeasurement, Gradient and Washout. These states can be requested from the SprayQc application and reacted to by the plugins.
MeasurementState GetMeasurementState();
Adds a message to the message box of the SprayQc window. This will not notify the operator.
void AddMessage(MessageType type, string message);
This method can be used to trigger a message to the operator and as such should be used sparingly. The data supplied in the parameters and
the functionality in the IRawFilename implementation is used to make up the message and retrieve the recipient.
void Notify(string subject, string message, string[] attachmentFiles, IRawFilename rawFile);
This method can be used to trigger a system-wide message that a catastrophic error has occurred which prevents the measurements from
continuing (as the Notify function, this should be used very sparingly). This will cause other plugins to shutdown operation of their
devices (like the column oven to prevent drying out of the column).
void OnCatastrophicError(CatastrophicError error);
This method can be called when a new raw-file has been detected. This is not necessarily part of the measurement management plugin tasks.
void MeasurementPending(IRawFilename rawFile);
These methods control the measurement progress / state updates of the SprayQc application and can only be called by a plugin that has taken this role.
void SetMeasurementState(MeasurementState state, IPlugin plugin);
void MeasurementStart(IRawFilename rawFile, IPlugin plugin);
void MeasurementFinish(IRawFilename rawFile, IPlugin plugin);
These methods have been provided for plugins that combine the functionality of multiple plugins. With these calls, the pointer to the appropriate plugins can be retrieved.
List<IPlugin> GetPlugins();
IPlugin GetPluginOfType(Type type);
|
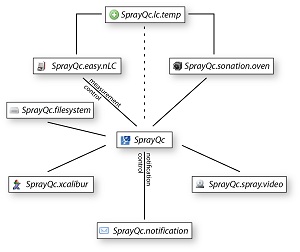
|